4 min to read
Java File Class
File Class 사용
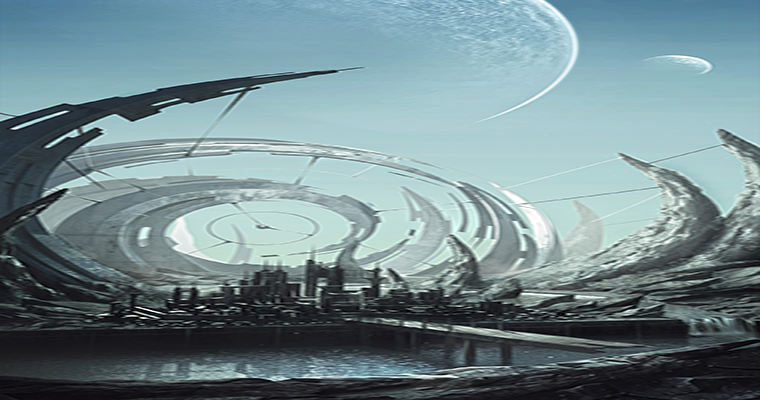
File Class
- 윈도우 에서는 디렉토리 구분자를 \\ 또는 / 로 구분한다.
- File 객체를 생성했다고 해서 파일이나 디렉토리가 생성되는 것은 아니다.
- exists() 메소드를 활용해 파일 이나 디렉토리가 있는지 없는지 확인 해야한다.
- exists() 메소드는 해당 디렉토리에 파일이나 디렉토리가 존재한다면 true 아니면 false를 리턴한다.
boolean isExist = file.exists();
- 위 isExist가 false 라면 아래 메소드로 파일 또는 디렉토리를 생성하면 된다.
리턴 타입 | 메소드 | 설명 |
---|---|---|
boolean | createNewFile() | 새로운 파일을 생성 |
boolean | mkdir() | 새로운 디렉토리를 생성 |
boolean | mkdirs() | 경로상에 없는 모든 디렉토리를 생성 |
boolean | delete() | 파일 또는 디렉토리 삭제 |
- 파일 또는 디렉토리가 존재할 경우에는 다음 메소드를 통해 정보를 얻어낼 수 있다.
리턴 타입 | 메소드 | 설명 |
---|---|---|
boolean | canExecute() | 실행할 수 있는 파일인지 여부 |
boolean | canRead() | 읽을 수 있는 파일인지 여부 |
boolean | canWrite() | 수정 및 저장할 수 있는 파일인지 여부 |
String | getName() | 파일의 이름을 리턴 |
String | getParent() | 부모 디렉토리를 리턴 |
File | getParentFile() | 부모 디렉토리를 File 객체로 생성 후 리턴 |
String | getPath() | 전체 경로를 리턴 |
boolean | isDirectory() | 디렉토리인지 여부 |
boolean | isFile() | 파일인지 여부 |
boolean | isHidden() | 숨김 파일인지 여부 |
long | lastModified() | 마지막 수정 날짜 및 시간을 리턴 |
long | length() | 파일의 크기를 리턴 |
String[] | list() | 디렉토리에 포함된 파일 및 서브디렉토리 목록 전부를 String 배열로 리턴 |
String[] | list(FilenameFilter filter) | 디렉토리에 포함된 파일 및 서브디렉토리 목록 중에 FillenameFilter에 맞는 것만 String 배열로 리턴 |
File[] | listFiles() | 디렉토리에 포함된 파일 및 서브 디렉토리 목록 전부를 File 배열로 리턴 |
File[] | listFiles(FilenameFilter filter) | 디렉토리에 포함된 파일 및 서브디렉토리 목록 중에 FilenameFilter에 맞는 것만 File 배열로 리턴 |
- 다음은 C:\Temp 디렉토리에 Dir 디렉토리와 file1.txt, file2.txt, file3.tx 파일을 생성하고, Temp 디렉토리에 있는 파일 목록을 출력하는 예제이다.
import java.io.File;
import java.net.URI;
import java.text.SimpleDateFormat;
import java.util.Date;
public class FileExam {
public static void main(String[] args) throws Exception{
String dir = "C:/Temp/Dir";
String file1 = "C:/Temp/file1.txt";
String file2 = "C:/Temp/file2.txt";
String file3 = "file:///C:/Temp/file3.txt";
File filePath = new File(dir);
File newFile1 = new File(file1);
File newFile2 = new File(file2);
File newFile3 = new File(new URI(file3)); // URI객체로 전달 가능.
if (!filePath.exists()) { filePath.mkdirs(); } // 디렉토리 가 없다면 디렉토리 생성
if (!newFile1.exists()) { newFile1.createNewFile(); } // 파일이 없다면 파일 생성
if (!newFile2.exists()) { newFile2.createNewFile(); }
if (!newFile3.exists()) { newFile3.createNewFile(); }
String pattern = "yyyy-MM-dd\ta\tHH:mm"; // 날짜 패턴 정의
File temp = new File("C:/Temp");
SimpleDateFormat sdf = new SimpleDateFormat(pattern);
File[] contents = temp.listFiles(); // temp경로의 파일 및 폴더 정보를 가져옴
System.out.println("날짜\t\t시간\t\t형태\t\t크기\t이름");
System.out.println("--------------------------------------------------------------------------");
for (File file : contents) { // for each 으로 파일 및 폴더 정보를 출력 시킴
// 마지막 수정 날짜를 Date로 객체 생성후
// SimpleDateFormat 클래스로 위 패턴에 맞게 날짜를 포맷시킴
System.out.print(sdf.format(new Date(file.lastModified())));
if(file.isDirectory()) { // file이 디렉토리면
System.out.print("\t<DIR>\t\t\t" + file.getName()); // 디렉토리 표시
} else { // file이 디렉토리가 아니면
System.out.print("\t\t\t" + file.length() + "\t" + file.getName()); // 파일 크기 및 이름 표시
}
System.out.println(); // 줄 개행 (라인피드 + 캐리지 리턴)
}
}
}
Comments