5 min to read
Java 함수적 인터페이스 Function
함수적 인터페이스의 사용
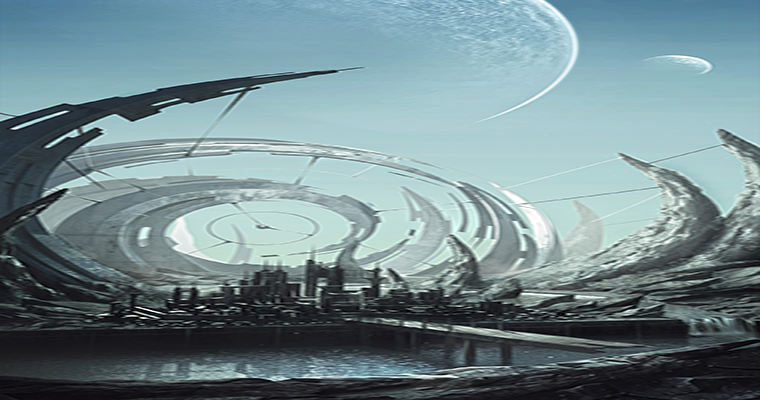
인터페이스명 | 추상메소드 | 설명 |
---|---|---|
Function<T, R> | R apply(T t) | 객체 T를 객체 R로 매핑 |
BiFunction<T, U, R> | R apply(T t, U u) | 객체 T와 U를 객체 R로 매핑 |
DoubleFunction |
R apply(double value) | double를 객체 R로 매핑 |
IntFunction |
R apply(int value) | int를 객체 R로 매핑 |
IntToDoubleFunction | double applyAsDouble(int value) | int를 double로 매핑 |
IntToLongFunction | long applyAsLong(int value) | int를 long으로 매핑 |
LongToDoubleFunction | double applyAsDouble(long value) | long을 double로 매핑 |
LongToIntFunction | int applyAsInt(long value) | long을 int로 매핑 |
ToDoubleBiFunction<T, U> | double applyAsDouble(T t, U u) | 객체 T와 U를 double로 매핑 |
ToDoubleFunction |
double applyAsDOuble(T t) | 객체 T를 double로 매핑 |
ToIntBiFunction<T, U> | int applyAsInt(T t, U u) | 객체 T와 U를 int로 매핑 |
ToIntFunction |
int applyAsInt(T t) | 객체 T를 int로 매핑 |
ToLongBiFunction<T, U> | long applyAsLong(T t, U u) | 객체 T와 U를 long으로 매핑 |
ToLOngFunction |
long applyAsLong(T t) | 객체 T를 long으로 매핑 |
Function Exam
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.function.ToIntFunction;
// JS 기본 문법
// https://opentutorials.org/course/3332/21030
public class FunctionExam {
private static List<Student> list = Arrays.asList(
new Student("홍길동", 90, 96),
new Student("신용권", 95, 93));
public static void printString( Function<Student, String> function ) {
for (Student student : list) { // list에 저장된 항목만큼 루핑시킴
System.out.print(function.apply(student) + " "); // 람다식 실행
}
System.out.println();
}
public static void printInt( ToIntFunction<Student> function ) {
for (Student student : list) { // List에 저장된 항목 수만큼 루핑
System.out.print(function.applyAsInt(student) + " "); // 람다식 실행
}
System.out.println();
}
public static void main(String[] args) {
System.out.println("[학생 이름]");
printString( t -> t.getName() );
System.out.println("[영어 점수]");
printInt(t -> t.getEngScore());
System.out.println("[수학 점수]");
printInt(t -> t.getMathScore());
}
}
Function Exam 2
import java.util.Arrays;
import java.util.List;
import java.util.function.ToIntFunction;
public class FunctionExam2 {
private static List<Student> list = Arrays.asList( // List를 만듦
new Student("홍길동", 90, 96),
new Student("신용권", 95, 93));
public static double avg( ToIntFunction<Student> function ) {
int sum = 0;
for (Student student : list) { // list에서 stu객체를 뽑아옴
sum += function.applyAsInt(student); // sum 변수에 누적시킴
}
double avg = (double) sum / list.size(); // 평균 구함
return avg;
}
public static void main(String[] args) {
double engListAvg = avg( s -> s.getEngScore() );
System.out.println("영어 점수 평균 : " + engListAvg);
double mathAvg = avg( s -> s.getMathScore() );
System.out.println("수학 평균 점수 : " + mathAvg);
}
}
Student Class
public class Student {
private String name;
private int engScore;
private int mathScore;
public Student(String name, int engScore, int mathScore) {
this.name = name;
this.engScore = engScore;
this.mathScore = mathScore;
}
public String getName() {
return name;
}
public int getEngScore() {
return engScore;
}
public int getMathScore() {
return mathScore;
}
}
Comments