2 min to read
Java Lambda(람다)식 사용
Lambda사용을 통해 함수적 인터페이스 를 이해한다.
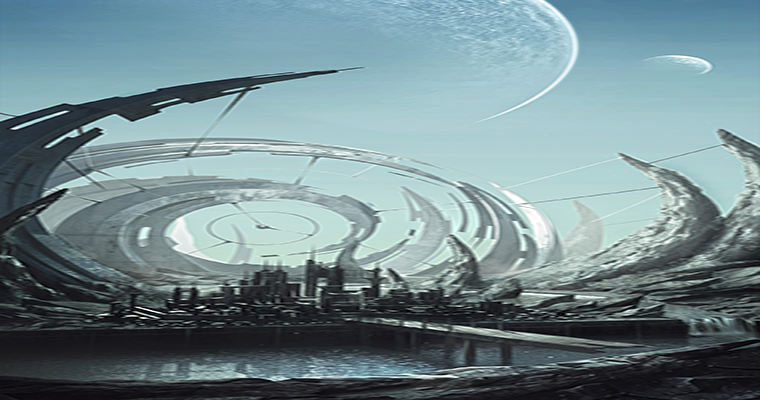
Lambda Main
public class Main {
public static void main(String[] args) {
// 매개값과 리턴 값 이 없는 함수적 인터페이스
MyFunctionInterface01 fi;
fi = () -> {
String str = "Method call1";
System.out.println(str);
};
fi.method();
// 두 번째 방법
fi = () -> { System.out.println("Method call2"); };
fi.method();
// 세 번째 방법
fi = () -> System.out.println("Method call3");
fi.method();
//=================================================
InterfaceUseVal iuv; // 매개변수가 하나 이상인 함수인터페이스
iuv = (x, y) -> { // 변수 x를 대입
int result = (x*y);
System.out.println(result);
};
iuv.method(5, 5);
// 혹은
iuv = (x, y) -> System.out.println(x*y);
iuv.method(4, 5);
//=================================================
InterfaceUseReturn iur; // 리턴값이 있는 인터페이스
// 첫 번째 방법
iur = (x, y) -> x + y;
int result = iur.method(5, 7);
System.out.println(result);
// 두 번째 방법
iur = (x, y) -> {
int result2;
result2 = x+y;
return result2;
};
System.out.println(iur.method(10, 30));
}
}
아래는 람다식 사용을 위한 인터페이스 구현 방법 3가지
// 두개이상의 추상메소드 선언을 금하게 해줌
// 함수적 인터페이스는 하나의 메소드만 선언 되어 있어야한다.
// 람다식이 하나의 메소드를 정의하기 때문에 두개 이상의 추상 메소드가 선언된
// 인터페이스는 람다식을 이용해서 구현 객체를 생성할 수 없다.
/* 매개값, 리턴값이 없는 인터페이스 */
@FunctionalInterface // 두새이상의 추상메소드 가 선언되지 않도록 어노테이션 으로 컴파일러가 확인하게끔 해준다.
public interface MyFunctionInterface01 {
public void method();
}
/* 매개값이 2개 이상인 인터페이스 */
@FunctionalInterface
public interface InterfaceUseVal {
public void method(int x, int y);
}
/* 매개값, 리턴값이 존재하는 인터페이스 */
@FunctionalInterface
public interface InterfaceUseReturn {
// 리턴값이 존재 하기 때문에 반환형을 선언 해 주어야 한다.
public int method(int x, int y);
}
Comments