13 min to read
R-Studio 정규식의 사용 Part.2
R-Studio의 강력한 정규식을 이용한 데이터제어
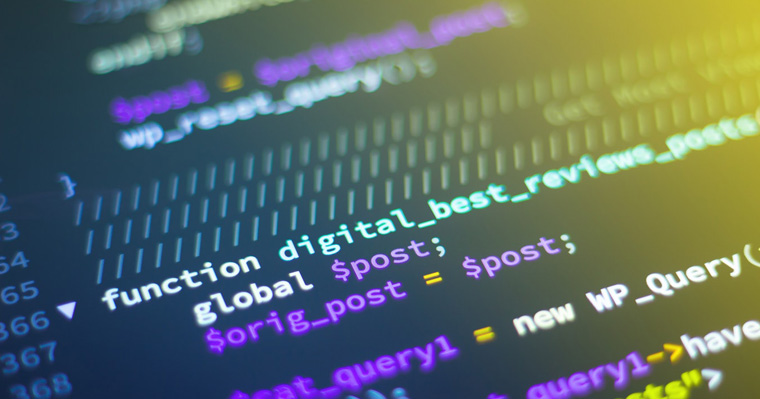
R-Studio 의 정규식
데이터의 필터를 위한 강력한 정규식 표현
Use | Descriptions |
---|---|
\\d |
숫자 |
\\D |
숫자가 아닌 것 |
\\s |
공백 |
\\S |
공백이 아닌 것 |
\\w |
단어 |
\\W |
단어가 아닌 것 |
\\t |
Tab |
\\n |
New Line (엔터 문자) |
^ |
시작되는 글자 |
$ |
마지막 글자 |
\ |
Escape Character (탈출 문자) |
| |
두 개 이상의 조건 (OR) |
[a-z] |
영어 소문자 |
[A-z] |
모든 영문자 |
i+ |
i가 최소 1회는 나오는 경우 |
i* |
i가 최소 0회 이상 나오는 경우 |
i? |
i가 최소 0회에서 최대 1회만 나오는 경우 (optional) |
i{n} |
i가 연속적으로 n회 나오는 경우 |
i{n1,n2} |
i가 n1에서 n2회 나오는 경우 |
i{n,} |
i가 n회 이상 나오는 경우 ’ , ‘ 뒤의 공백 유의 |
[:alnum:] |
문자와 숫자가 나오는 경우 : [:alpha:] and [:digit:] |
[:alpha:] |
문자가 나오는 경우 : [:lower:] and [:upper:] |
[:blank:] |
공백이 있는 경우 |
[:cntrl:] |
제어문자가 있는 경우 |
[:digit:] |
0 ~ 9 |
[:graph:] |
Graphical characters : [:alnum:] and [:punct:] |
[:lower:] |
소문자가 있는 경우 |
[:print:] |
숫자, 문자, 특수문자, 공백 모두 |
[:space:] |
공백문자 |
[:upper:] |
대문자가 있는 경우 |
[:xdigit:] |
16진수가 있는 경우 |
함수 예제
myString <- "hello1234world5678"
str_extract(myString, "[0-9]{2}")
str_extract_all(myString, "[0-9]{2}")
# 숫자가 최소 3개 이상
str_extract_all(myString, "[0-9]{3,}")
# 숫자 3개 뽑아옴
str_extract_all(myString, "[0-9]{3}")
# 문자 3~4자 만 뽑아옴
myString2 <- "abc이순신cd1235이다도시world5678"
str_extract_all(myString2, "[가-히]{3,4}")
# 공백을 기준으로 문자열 뽑아옴
myString3 <- "abc123홍길동 def"
# 공백을 토큰으로 3자 이상 문자열을 list로 뽑아옴
str_extract_all(myString3, "\\w{3,}")
# 공백을 기준으로 4자 이상 문자열 뽑아옴
str_extract_all(myString3, "\\w{4,}")
# email 정규식 조합
email <- "abcd@naver.com;def@daum.net"
# @앞 3자리이상, @ 뒤 3자리이상에 .이 붙고 .다음에 2자리 이상.
regEx <- "\\w{3,}@\\w{3,}.\\w{2,}"
str_extract_all(email, regEx)
# email 정규식 조합2
# 1번째 글자는 반드시 알파벳으로 시작해야 한다.
# id는 4글자 이상이어야 한다.
email <- "abcd@naver.com;1def@daum.net"
regEx <- "[a-z]{1}\\w{3,}@\\w{3,}.\\w{2,}"
str_extract_all(email, regEx)
# 주민번호 솎아내기
# 13자리 또는 14자리
# 뒷자리 처음에는 1234중에 하나이다.
idCode <- "700828-1234111 600828-1234222 500828-6234333 400828123444"
# regEx <- "[0-9]{6}-?[1234][0-9]{6}"
regEx <- '\\d{6}-?[1-4]\\d{6}'
str_extract_all(idCode, regEx)
# 벡터 문자열중 필요한 문자열 혹은 문자 단위 를 검색해 bool 리턴
beverage <- c("cola", "fanta", "Cola", "serverup", "orange")
beverage
# C를 beverage 벡터에서 포함된 문자열 을 찾음. 찾으면 T 아니면 F로 리턴시킴
test01 <- str_detect(beverage, 'C')
test01
beverage[test01]
# 소문자 c있는 벡터 T
str_detect(beverage, "^c")
# 대소문자 구별 없이 c 벡터 T
str_detect(beverage, "^[cC]")
# 소문자 a로 끝나는 문자열 찾기
str_detect(beverage, "a$")
# 소문자 p나 e로 끝나는 거 찾기
str_detect(beverage, "[pe]$")
# n 있는거 찾음
str_detect(beverage, "n")
str_detect(beverage, "[n]")
# 특정한 단어를 카운트 해줌
myCount <- str_count(beverage, "[a]")
myCount
myCount <- str_count(beverage, "[af]")
myCount
# 요소별로 100번 추가시킴
dup01 <- str_dup(beverage, 100)
dup01
# 문자열 양 끝 공백 다 지워줌
string8 <- " col fanta "
trim01 <- str_trim(string8, side="both")
trim01
# 문자열 왼쪽만 공백 다 지워줌
str_trim(string8, side="left")
콘솔 결과
> myString <- "hello1234world5678"
> str_extract(myString, "[0-9]{2}")
[1] "12"
> str_extract_all(myString, "[0-9]{2}")
[[1]]
[1] "12" "34" "56" "78"
>
> # 숫자가 최소 3개 이상
> str_extract_all(myString, "[0-9]{3,}")
[[1]]
[1] "1234" "5678"
>
> # 숫자 3개 뽑아옴
> str_extract_all(myString, "[0-9]{3}")
[[1]]
[1] "123" "567"
>
> # 문자 3~4자 만 뽑아옴
> myString2 <- "abc이순신cd1235이다도시world5678"
> str_extract_all(myString2, "[가-히]{3,4}")
[[1]]
[1] "이순신" "이다도시"
>
> # 공백을 기준으로 문자열 뽑아옴
> myString3 <- "abc123홍길동 def"
> # 공백을 토큰으로 3자 이상 문자열을 list로 뽑아옴
> str_extract_all(myString3, "\\w{3,}")
[[1]]
[1] "abc123홍길동" "def"
> # 공백을 기준으로 4자 이상 문자열 뽑아옴
> str_extract_all(myString3, "\\w{4,}")
[[1]]
[1] "abc123홍길동"
>
> # email 정규식 조합
> email <- "abcd@naver.com;def@daum.net"
> # @앞 3자리이상, @ 뒤 3자리이상에 .이 붙고 .다음에 2자리 이상.
> regEx <- "\\w{3,}@\\w{3,}.\\w{2,}"
> str_extract_all(email, regEx)
[[1]]
[1] "abcd@naver.com" "def@daum.net"
>
> # email 정규식 조합2
> # 1번째 글자는 반드시 알파벳으로 시작해야 한다.
> # id는 4글자 이상이어야 한다.
> email <- "abcd@naver.com;1def@daum.net"
> regEx <- "[a-z]{1}\\w{3,}@\\w{3,}.\\w{2,}"
> str_extract_all(email, regEx)
[[1]]
[1] "abcd@naver.com"
>
> # 주민번호 솎아내기
> # 13자리 또는 14자리
> # 뒷자리 처음에는 1234중에 하나이다.
> idCode <- "700828-1234111 600828-1234222 500828-6234333 400828123444"
> # regEx <- "[0-9]{6}-?[1234][0-9]{6}"
> regEx <- '\\d{6}-?[1-4]\\d{6}'
> str_extract_all(idCode, regEx)
[[1]]
[1] "700828-1234111" "600828-1234222"
>
> # 벡터 문자열중 필요한 문자열 혹은 문자 단위 를 검색해 bool 리턴
> beverage <- c("cola", "fanta", "Cola", "serverup", "orange")
> beverage
[1] "cola" "fanta" "Cola" "serverup" "orange"
>
> # C를 beverage 벡터에서 포함된 문자열 을 찾음. 찾으면 T 아니면 F로 리턴시킴
> test01 <- str_detect(beverage, 'C')
> test01
[1] FALSE FALSE TRUE FALSE FALSE
> beverage[test01]
[1] "Cola"
>
> # 소문자 c있는 벡터 T
> str_detect(beverage, "^c")
[1] TRUE FALSE FALSE FALSE FALSE
>
> # 대소문자 구별 없이 c 벡터 T
> str_detect(beverage, "^[cC]")
[1] TRUE FALSE TRUE FALSE FALSE
>
> # 소문자 a로 끝나는 문자열 찾기
> str_detect(beverage, "a$")
[1] TRUE TRUE TRUE FALSE FALSE
>
> # 소문자 p나 e로 끝나는 거 찾기
> str_detect(beverage, "[pe]$")
[1] FALSE FALSE FALSE TRUE TRUE
>
> # n 있는거 찾음
> str_detect(beverage, "n")
[1] FALSE TRUE FALSE FALSE TRUE
> str_detect(beverage, "[n]")
[1] FALSE TRUE FALSE FALSE TRUE
>
> # 특정한 단어를 카운트 해줌
> myCount <- str_count(beverage, "[a]")
> myCount
[1] 1 2 1 0 1
>
> myCount <- str_count(beverage, "[af]")
> myCount
[1] 1 3 1 0 1
>
> # 요소별로 100번 추가시킴
> dup01 <- str_dup(beverage, 100)
> dup01
[1] "colacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacolacola"
[2] "fantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafantafanta"
[3] "ColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaColaCola"
[4] "serverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverupserverup"
[5] "orangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorangeorange"
>
> # 문자열 양 끝 공백 다 지워줌
> string8 <- " col fanta "
> trim01 <- str_trim(string8, side="both")
> trim01
[1] "col fanta"
>
> # 문자열 왼쪽만 공백 다 지워줌
> str_trim(string8, side="left")
[1] "col fanta "
Comments